Two-Dimensional Grid Movement¶
This example shows how to perform two-dimensional grid movement using two devices. One device is for X axis and second for Y axis. Goal is to visit predefined points within the grid.
The movement is performed on grid of size 40 x 20 mm. First point is on coordinates x=2 mm, y=2 mm. Step on X axis is 5 mm and on axis is Y 4 mm respectively. See picture below.
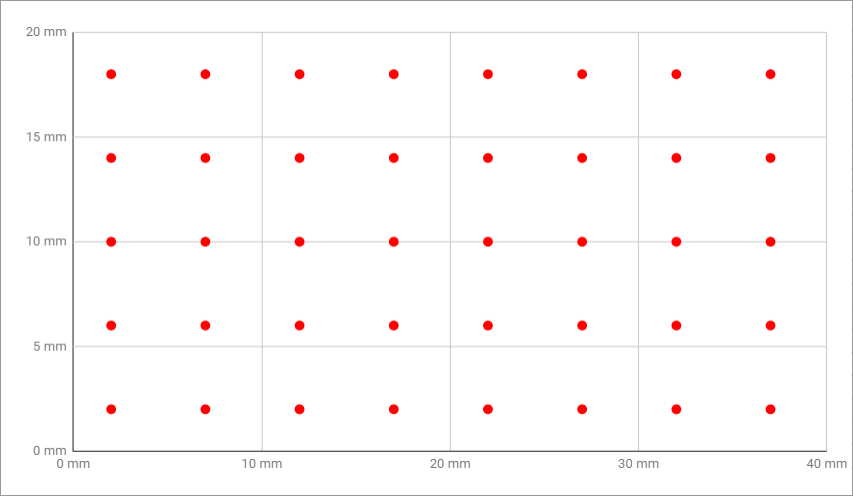
Program first homes both axis and the moves through all the points. At the end both axis are homed again.
Program stops for 1 second on each point and prints its coordinates. You can always stop program by pressing Ctrl+C
.
Before you begin with code you should use Zaber Console to connect to port and identify both devices. Make sure to change the port within code to appropriate one:
# choose appropriate port on your computer (currently COM5)
SERIAL_PORT = "COM5"
It is important that devices are properly numbered (have correct numbers assigned). Renumbering of devices can be performed using Zaber Console. Examples assumes that X-axis device has number 1 and Y-axis has number 2.
# assuming the X axis device has number 1 and Y axis device has number 2
DEVICE_X_NUMBER = 1
DEVICE_Y_NUMBER = 2
Example is using mathematical conversion between device specific microsteps and millimeters. Correct conversion results depend on right value of constants:
# device specific values used to convert distance to microsteps
DEVICE_X_MICROSTEP_SIZE_MICROMETERS = 0.124023437 # micrometers
DEVICE_Y_MICROSTEP_SIZE_MICROMETERS = 0.1905 # micrometers
Please set appropriate values depending on what devices are used. Values can be found in devices’ datasheets as “Microstep Size (Default Resolution)”.
Example code (Download
):
import time
from zaber.serial import AsciiSerial, AsciiDevice
# choose appropriate port on your computer (currently COM5)
SERIAL_PORT = "COM5"
# assuming the X axis device has number 1 and Y axis device has number 2
DEVICE_X_NUMBER = 1
DEVICE_Y_NUMBER = 2
# device specific values used to convert distance to microsteps
DEVICE_X_MICROSTEP_SIZE_MICROMETERS = 0.124023437 # micrometers
DEVICE_Y_MICROSTEP_SIZE_MICROMETERS = 0.1905 # micrometers
def main():
# create and open serial port
port = AsciiSerial(SERIAL_PORT)
# instantiate device for x-axis and y-axis
device_x = AsciiDevice(port, DEVICE_X_NUMBER)
device_y = AsciiDevice(port, DEVICE_Y_NUMBER)
# home devices at the beginning
device_x.home()
device_y.home()
# X axis - start at 2mm, move till 40mm (non-inclusive) with 5mm step
for x_mm in range(2, 40, 5):
# convert position on X axis to device microsteps (integer number)
x_microsteps = int(x_mm * 1000 / DEVICE_X_MICROSTEP_SIZE_MICROMETERS)
# order device to move to that position
device_x.move_abs(x_microsteps)
# Y axis - start at 2mm, move till 20mm (non-inclusive) with 4mm step
for y_mm in range(2, 20, 4):
# convert position on Y axis to device microsteps (integer number)
y_microsteps = int(y_mm * 1000 / DEVICE_Y_MICROSTEP_SIZE_MICROMETERS)
# order device to move to that position
device_y.move_abs(y_microsteps)
# print current position
print("Position: x={}mm y={}mm".format(x_mm, y_mm))
# wait 1 second before next move
time.sleep(1)
# home devices at the end
device_x.home()
device_y.home()
# run main function on program start
if __name__ == '__main__':
main()